Background
I woke up this Christmas morning to find out that our Mailgun account had sent over 500 messages in an hour, and our account was suspended. Since the account in question is still under development, the chances of a runaway script are high.. but before I did any fixing, I needed to see what messages were sent, to whom and when. I was able to get a list of messages using the Mailgun API, but since the response included the timestamp of when the message was sent, I needed to format the timestamp field so I could see the date and time of the event.
Pull the relevant data from Mailgun
First, I needed to get a list of messages that were sent via Mailgun.. since the system is still in development, I don’t have an internal logging mechanism that would tell me this easily. So, I resorted to the Mailgun API, specifially the Events API.
Since I use Postman, and they have a Postman collection already, the query was made to the following url.
https://api.mailgun.net/v3/{{mydomain}}/events?event=accepted
Analyze the data in Postman
The response from Mailgun looks something like this.. (actual data has been redacted)
{
"items": [
{
"envelope": {
"targets": "[email protected]",
"transport": "smtp",
"sender": "postmaster@mydomain"
},
"timestamp": 1703538675.2227187,
"log-level": "info",
"flags": {
"is-test-mode": false,
"is-authenticated": true
},
...
},
{
"envelope": {
"targets": "[email protected]",
"transport": "smtp",
"sender": "postmaster@mydomain"
},
"timestamp": 1703538675.2227187,
"log-level": "info",
"flags": {
"is-test-mode": false,
"is-authenticated": true
},
...
},
]
}
Visualize the data in Postman
I asked Postbot to give me visualize this for me in a table and the timestamp column came out looking like this:
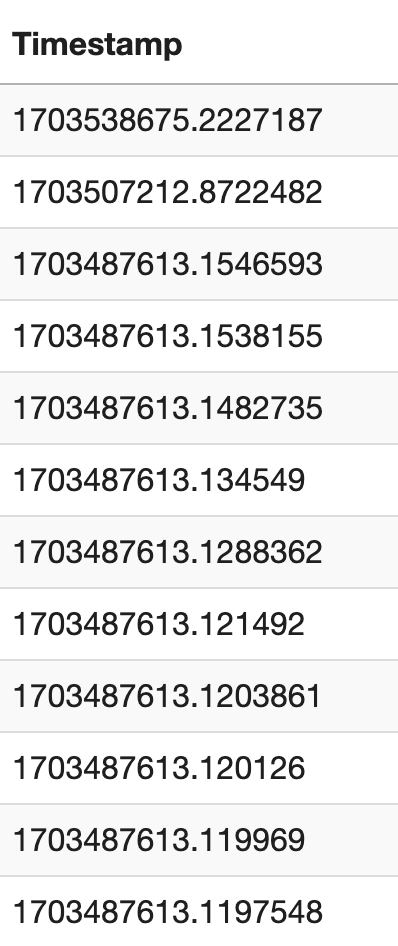
Formatting the timestamp field
I had to format the timestamp field, so I could see the date and time of the event. I used the following code to format the timestamp field.
const responseData = pm.response.json();
responseData.items.forEach(function(item) {
if (item.timestamp) {
// Convert Unix timestamp to a Date object and format it
item.timestamp = new Date(item.timestamp * 1000).toLocaleString();
}
});
pm.visualizer.set(template, { response: responseData.items });
Which resulted in the following table:
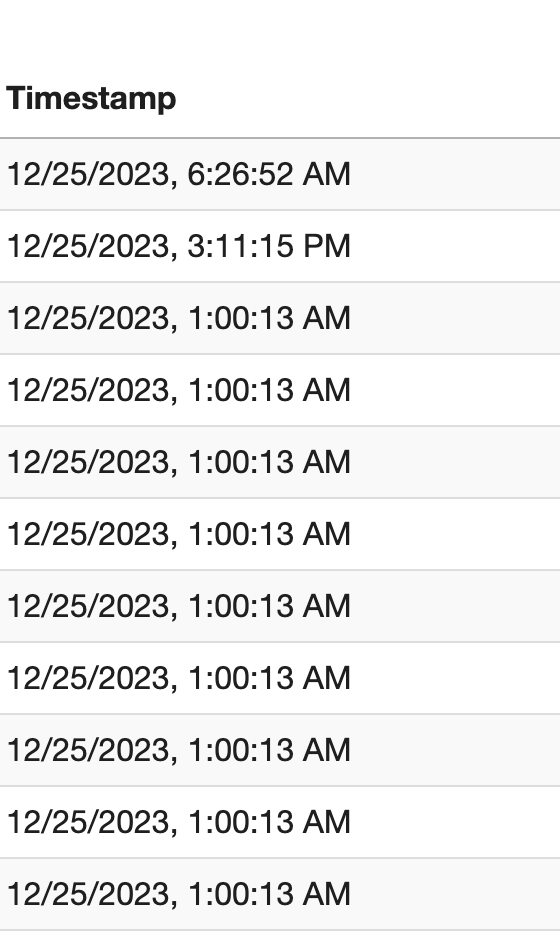
Conclusion
Now, I can run this query and see what messages were sent, to whom and when. I can also see if there are any patterns in the data that would indicate a runaway script.